Server API¶
Data Containers¶
Core¶
The following are the underlying, lowest-level classes for holding data which can be sent over an EPICS V3 channel in caproto.
Numeric Data¶
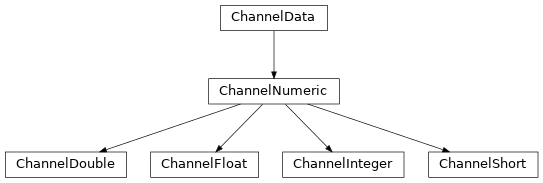
|
Base class holding data and metadata which can be sent across a Channel. |
|
64-bit floating point data and metadata which can be sent over a channel. |
|
32-bit floating point data and metadata which can be sent over a channel. |
|
32-bit LONG integer data and metadata which can be sent over a channel. |
|
16-bit SHORT integer data and metadata which can be sent over a channel. |
String or Byte Data¶
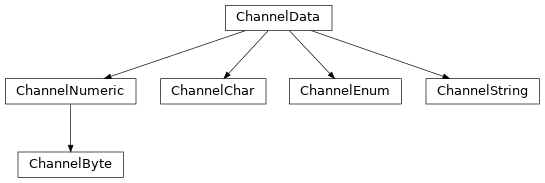
|
8-bit unencoded CHAR data plus metadata which can be sent over a channel. |
|
8-bit encoded CHAR data plus metadata which can be sent over a channel. |
|
ENUM data which can be sent over a channel. |
|
8-bit encoded string data plus metadata which can be sent over a channel. |
Base Class¶
The above share methods from their common (internal) base class
ChannelData
.
|
Base class holding data and metadata which can be sent across a Channel. |
PvpropertyData¶
pvproperty builds on top of ChannelData with the following classes, separated by whether or not they are read-only.
|
A top-level class for mixing in with ChannelData subclasses. |
Read/write¶

Read-write ENUM data for pvproperty, with Off/On as default strings. |
|
|
Read-write 8-bit CHAR data for pvproperty for bytes. |
|
Read-write 8-bit CHAR data for pvproperty with string encoding. |
|
Read-write DOUBLE data for pvproperty (64 bits). |
|
Read-write ENUM data for pvproperty. |
|
Read-write FLOAT data for pvproperty (32 bits). |
|
Read-write LONG data for pvproperty (32 bits). |
A mixin class which marks this data as read-only from channel access. |
|
|
Read-write SHORT/INT data for pvproperty (16 bits). |
|
Read-write STRING data for pvproperty (up to 40 chars). |
Read-only¶
Read-only data classes mix in with
PvpropertyReadOnlyData
.

Read-only ENUM data for pvproperty, with Off/On as default strings. |
|
|
Read-only 8-bit CHAR data for pvproperty for bytes. |
|
Read-only 8-bit CHAR data for pvproperty with string encoding. |
|
Read-only DOUBLE data for pvproperty (64 bits). |
|
Read-only ENUM data for pvproperty. |
|
Read-only FLOAT data for pvproperty (32 bits). |
|
Read-only LONG data for pvproperty (32 bits). |
|
Read-only SHORT/INT data for pvproperty (16 bits). |
|
Read-only STRING data for pvproperty (up to 40 chars). |
High-level API / pvproperty magic¶
|
Create new instance of PVSpec(get, put, startup, shutdown, attr, name, dtype, value, max_length, alarm_group, read_only, doc, fields, scan, record, cls_kwargs) |
|
Expand a PV name with Python {format-style} macros |
|
Class which groups a set of PVs for a high-level caproto server |
|
A property-like descriptor for specifying a PV in a PVGroup. |
|
A top-level class for mixing in with ChannelData subclasses. |
|
A property-like descriptor for specifying a subgroup in a PVGroup. |
|
A descriptor for making an RPC-like function. |
|
Expand a PV name with Python {format-style} macros |
Generates a Subgroup class for a pair of PVs (setpoint and readback). |
|
|
Wrap a function intended for pvproperty.scan with common logic to periodically call it. |
Command-line Helpers¶
Tools for helping to generate command-line argument parsers for use with IOCs.
|
Construct a template arg parser for starting up an IOC |
|
A reusable ArgumentParser for basic example IOCs. |
|
Run an IOC, given its PV database dictionary and async-library module name. |
Advanced / Internal¶
The following are related to the internal mechanisms that PVGroup and pvproperty rely on.
|
A proxy class which allows access to |
|
A field specification for a pvproperty record. |
Nested pvproperty which allows decorator usage in parent class |
|
|
Metaclass that finds all pvproperties |
Library-agnostic server core¶
Core Classes¶
These are mostly as-is by all server implementations.
Library compatibility layer |
|
|
Create new instance of Subscription(mask, channel_filter, circuit, channel, data_type, data_count, subscriptionid, db_entry) |
Create new instance of SubscriptionSpec(db_entry, data_type_name, mask, channel_filter) |
Base Classes¶
These are intended to be subclassed to implement support for a new async library.
|
|
asyncio server¶
Implementation classes¶
Classes built on top of the library-agnostic core, used to implement asyncio functionality.
|
|
|
Implement the |
Wraps a caproto.VirtualCircuit with an asyncio client. |
Helper functions¶
|
Run an IOC, given its PV database dictionary. |
|
Start an asyncio server with a given PV database |
Curio server¶
Implementation classes¶
Classes built on top of the library-agnostic core, used to implement asyncio functionality.
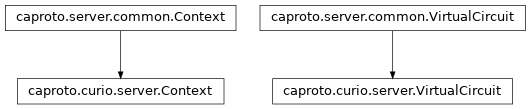
|
|
|
Wraps a caproto.VirtualCircuit with a curio client. |
Helper functions¶
|
Run an IOC, given its PV database dictionary. |
|
Start a curio server with a given PV database |
Miscellaneous¶
Trio server¶
Implementation classes¶
Classes built on top of the library-agnostic core, used to implement asyncio functionality.
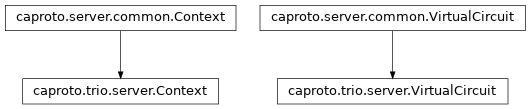
|
|
The class wraps trio.Event and implements Event.clear() method that is used in the code, but deprecated in trio. |
|
|
Wraps a caproto.VirtualCircuit with a trio client. |
Helper functions¶
|
Run an IOC, given its PV database dictionary. |
|
Start a trio server with a given PV database |